# Custom Response Validator Or Operator
In this guide, we will learn how you may define your own custom response validator or custom assertion operator in vREST NG Application. Custom Validator validates the actual response in your own way. Most of the cases of response validation can be handled with the default validators. However, you may define your own custom validators for customized response validation scenarios.
There are majorly two scenarios in which this section will be helpful:
- If you want to perform custom response validation on full API responses. For such cases, use the
Text Body
assertions and leave theExpected Value
column blank. Instead set theExpected Body
. Then you will receive the full expected response usingthis.expectedResponse
inside the validator. - If you want to create a generic assertion operator which can be applied to partial API response as well. For such cases use any of the assertion sources except
Status Code
,Response Time
,Response Header
and you may also set theExpected Value
in such cases. You will receive the expected and actual value viathis.expectedValue
andthis.actualValue
respectively.
Now, let's see how you may add a custom response validator for your assertions. To create a custom response validator or operator,
- Go to Configuration Tab >> Response Validators section
- Click on the Add Response Validator button, to create the new response validator.
- Edit the validator code according to yourself.
- Give it a name and save it.
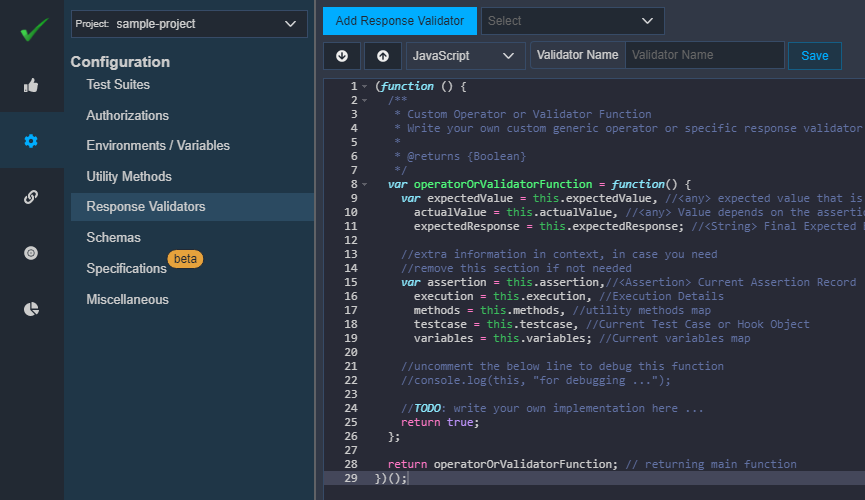
Let's see the various properties which are available via this
inside the validator function. The following properties are available:
expectedValue
actualValue
expectedResponse
assertion
execution
methods
testcase
variables
For more information about these properties and their purpose, please read our guide on Context Parameters.
And if you would like to debug the validator function then you may use standard console.log
statements to do that. For more information, please read our guide on Debugging.
Now, let's take a use case. Suppose we want to create a custom operator startsWith
that will make the assertion pass if the actual value starts with the value specified in the Expected Value
column.
For this purpose, first we will create a new Response Validator startsWith
as shown in the figure below:
You may use the following code to write this operator:
(function () {
/**
* Starts With Operator
* return true if the actualValue starts with the value specified in the `Expected Value` column
*
* @returns {Boolean}
*/
var startsWithFunction = function() {
var expectedValue = this.expectedValue, //<any> expected value which is set in the Assertions table
actualValue = this.actualValue; //<any> Value depends on the assertion source
return typeof expectedValue === 'string' && typeof actualValue === 'string' && actualValue.startsWith(expectedValue);
};
return startsWithFunction;
})();
Now let's see, how you may use this operator to validate your API responses. So, for this purpose, you may simply define any JSON body, XML Body, Text Body, Custom Source type assertion as per your need as shown in the figure below:
So, writing custom assertion operators in vREST NG is quite easy. With this, you may easily extend the vREST NG validation engine power.
# Response Validator Parameters:
WARNING
Deprecation Notice: However, we also provide these three parameters testcase
, response
, and methods
to response validators. But we recommend you to use the properties available on this
for this purpose. In the future, we will remove these parameters. And if you use the parameters available via this
then you will need not make any changes in the response validators in the future.
These deprecated parameters are explained below:
- testcase
The first parameter is the test case, which contains the information about the expected response.
response
The second parameter is the actual response of the HTTP request. It is a JSON object with the following keys:
- headers: HTTP Response headers retrieved
- actualResults: HTTP Response result retrieved. It is also a JSON object with the following keys:
- content: (String) Actual HTTP Response body
- resultType: (String) Content-type of HTTP Response body
methods
The third parameter is the methods object. This parameter is a JSON object where the key is the utility method name and the value is the utility method reference.
- So, you may write your own custom utility methods and use them in your custom response validators.