# Basic Response Validation
With the help of assertions and response validators, you can validate various response attributes like
- Status Code,
- Response Headers,
- Useful to validate the response header's values.
- Response Time,
- Useful to validate the API's performance. If an API is not returning response in a pre-defined time period then APIs implementation can be improved.
- Response Content (Text Body or JSON Body or XML Body)
- here Text body assertions can be applied to any type of response text content.
The screenshot below gives an idea of how vREST handles response validation.
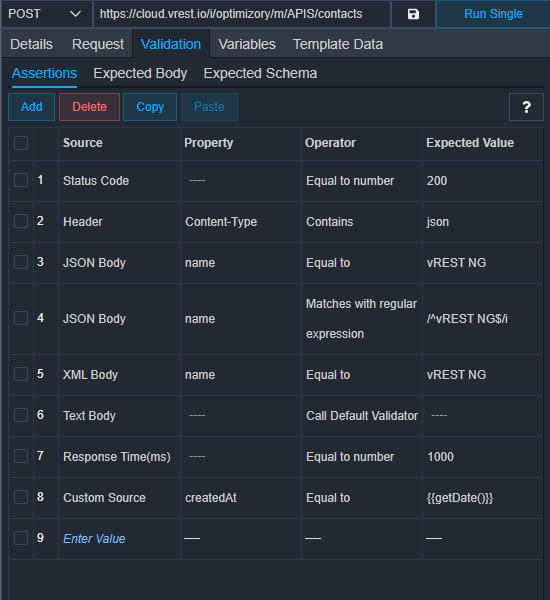
# Status Code
The most basic assertion that judges if the test case will pass or fail is the Status Code assertion. You can see in the above screenshot, how you can apply a Status Code assertion.
It provides 6 operators to apply as conditions:
- Equal to number: This allows you to check if a Status Code is equal to a given number, or not.
- Does not equal to number: This allows you to check if a Status Code is not equal to a given number, or not.
- Less than: This allows you to check if a Status Code is less than a given number, or not.
- Less than or equal to: This allows you to check if a Status Code is less than or equal to a given number, or not.
- Greater than: This allows you to check if a Status Code is greater than a given number, or not.
- Greater than or equal to: This allows you to check if a Status Code is greater than or equal to a given number, or not.
# Response Time
This assertion allows you to set certain conditions on the Response Time(ms) of your test case.
It also provides 6 operators to apply as conditions:
- Equal to number: This allows you to check if a Response Time is equal to a given number, or not.
- Does not equal to number: This allows you to check if a Response Time is not equal to a given number, or not.
- Less than: This allows you to check if a Response Time is less than a given number, or not.
- Less than or equal to: This allows you to check if a Response Time is less than or equal to a given number, or not.
- Greater than: This allows you to check if a Response Time is greater than a given number, or not.
- Greater than or equal to: This allows you to check if a Response Time is greater than or equal to a given number, or not.
# JSON Body
JSON Body assertion allows you to apply specific assertions on any part of a JSON response. In this assertion, you have to provide the property to track the JSON element. For eg, user.username
It provides the following operators to apply as conditions:
- Equal to: It checks if the value of the given property is equal to the expected value.
- Does not equal to: It checks if the value of the given property is not equal to the expected value.
- Is empty: It checks if the value of the given property is empty.
- Is not empty:It checks if the value of the given property is not empty.
- Contains: It checks if the value of the given property contains the expected value.
- Does not contain: It checks if the value of the given property does not contain the expected value.
- Matches with regular expression: It checks if the value of the given property matches with the expected value which can be entered as a regular expression. (Used in the case of dynamic values.)
- Is a number: It checks if the value of the given property is a number.
- Is a boolean: It checks if the value of the given property is a boolean.
- Equal to number: It checks if the value of the given property is equal to a given number.
- Does not equal to number: It checks if the value of the given property is not equal to given number.
- Less than: It checks if the value of the given property is less than the expected value.
- Less than or equal to: It checks if the value of the given property is less than or equal to the expected value.
- Greater than: It checks if the value of the given property is greater than the expected value.
- Greater than or equal to: It checks if the value of the given property is greater than or equal to the expected value.
- Has key: It checks if the value of the given property has a JSON key or not.
- Doesn't Have key: It checks if the value of the given property doesn't have the specified key.
- Has value: It checks if the value of the given property has a certain value assigned to a JSON key.
- Is null: It checks if the value of the given property is null.
# XML Body
XML Body assertion allows you to apply specific assertions on any part of a XML response. In this assertion you have to provide the property to track the XML element. For eg, user/username
It provides the following operators to apply as conditions:
- Equal to: It checks if the value of the given property is equal to the expected value.
- Does not equal to: It checks if the value of the given property is not equal to the expected value.
- Is empty: It checks if the value of the given property is empty.
- Is not empty:It checks if the value of the given property is not empty.
- Contains: It checks if the value of the given property contains the expected value.
- Does not contain: It checks if the value of the given property does not contain the expected value.
- Matches with regular expression: It checks if the value of the given property matches with the expected value which can be entered as a regular expression. (Used in the case of dynamic values.)
- Is a number: It checks if the value of the given property is a number.
- Is a boolean: It checks if the value of the given property is a boolean.
- Equal to number: It checks if the value of the given property is equal to a given number.
- Does not equal to number: It checks if the value of the given property is not equal to a given number.
- Less than: It checks if the value of the given property is less than the expected value.
- Less than or equal to: It checks if the value of the given property is less than or equal to the expected value.
- Greater than: It checks if the value of the given property is greater than the expected value.
- Greater than or equal to: It checks if the value of the given property is greater than or equal to the expected value.
- Has key: It checks if the value of the given property has a JSON key or not.
- Doesn't Have key: It checks if the value of the given property doesn't have the specified key.
- Has value: It checks if the value of the given property has a certain value assigned to a JSON key.
- Is null: It checks if the value of the given property is null.
# Text Body (Other types)
If your assertions are of any different type, then you can apply the Text Body assertion.
It provides 17 operators to apply as conditions:
- Equal to: It checks if the value of the given property is equal to the expected value.
- Does not equal to: It checks if the value of the given property is not equal to the expected value.
- Is empty: It checks if the value of the given property is empty.
- Is not empty:It checks if the value of the given property is not empty.
- Contains: It checks if the value of the given property contains the expected value.
- Does not contain: It checks if the value of the given property does not contain the expected value.
- Matches with regular expression: It checks if the value of the given property matches with the expected value which can be entered as a regular expression. (Used in the case of dynamic values.)
- Is a number: It checks if the value of the given property is a number.
- Is a boolean: It checks if the value of the given property is a boolean.
- Equal to number: It checks if the value of the given property is equal to a given number.
- Does not equal to number: It checks if the value of the given property is not equal to a given number.
- Less than: It checks if the value of the given property is less than the expected value.
- Less than or equal to: It checks if the value of the given property is less than or equal to the expected value.
- Greater than: It checks if the value of the given property is greater than the expected value.
- Greater than or equal to: It checks if the value of the given property is greater than or equal to the expected value.
Note: The other two operators, of Text Body assertion, comes under Advanced Response Validation.
# Custom Source
Custom Source is responsible for validating other sources e.g. Database state, file system state, etc. For more information, please visit Custom Source Validation