# Utility Methods
Similar to variables, in vREST, Utility Methods provide a way to dynamically change the test case properties at run time.
Utility Methods,
- provide dynamic values to test cases.
- are also available to Response Validators as a third argument.
Utility methods in vREST, can be accessed by using the format {{method_name(argument1, argument2, ...)}}
.
Similar to variables, utility methods can be used in:
- Request URL
- Request Parameter value
- Request Header value
- Request Body
- Response Assertions
- Property Column
- Expected Value Column
- Response Expected Body
- Response Expected Schema
- Variable Extractor Tab
- Authorization Configurations
Important Note:
- Inside a utility method, any utility method can be invoked by using
this.methods.METHOD_NAME.call(this, arg1, arg2, ...);
- Inside a utility method, any global/extracted variable can be accessed using
this.variables.VAR_NAME
. - If you use the utility method in Authorization configurations, then the current API request can be accessed by using
this.request
. - If you use the utility method in the Variable Extractor tab, then the current API execution can be accessed by using
this.execution
. - For more information on context parameters, please read this guide link.
- The code that is written inside the returning function in the utility method will be executed only when you invoke it somewhere in your API test and any other code that is written outside the returning function will be executed before the execution of API tests.
By Default, the following utility methods are available in vREST:
# getRandom
This method returns a random number of length 7 if no arguments are passed or return a random integer between min (included) and max (excluded). This method is also hidden.
# getDate
This method returns a date timestamp string if no arguments are passed or return the number of milliseconds between midnight of January 1, 1970, and the current date if true is passed as an argument. This method is also hidden.
# getHeader
This utility method can be used in the Variable Extractor tab and is helpful in extracting the value of a response header. This is useful in performing token-based authentication in vREST.
# number
This utility method can be used in converting a numeric string input into a number. If the input value type does not string then it returns the input value as it is.
# boolean
This utility method can be used in converting a string input ("true" / "false") into a boolean equivalent. If the input value type does not string then it returns the input value as it is.
# object
This utility method can be used in converting a JSON string input into a JSON object. If the input value type is not a string or not a valid JSON string then it returns the input value as it is.
# ifElse
This utility method can be used in returning values according to the defined condition. As of now, it is being used in defining some of the assertion result summary templates, but you may also use it for your own purposes.
# Custom Utility Methods
Apart from built-in utility methods, a user can create custom utility methods. To create a custom utility method,
- Go to Configuration Tab >> Utility Methods section.
- Click on
- Enter the code, Write a Method Name and Save the method.
Let's take some examples, how utility methods are useful in providing dynamic values to your test cases while testing.
# Example 1:
Suppose we have an API that accepts a parameter having a current timestamp. How you can provide values to such test cases? Utility Methods are a solution:
- First define a utility method in the Utility Methods section or use any built-in utility method like so
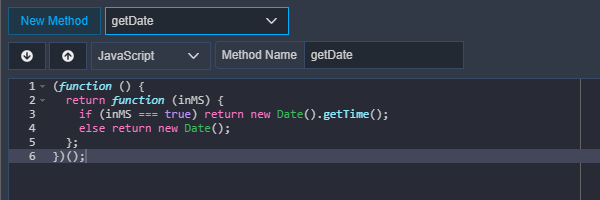
- Then use this utility method in your test case like this
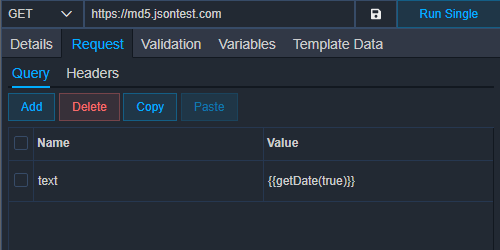
# Example 2:
Now, suppose we have a numeric variable say "counter" having an initial value say 1 (This initial value can be obtained from either global variable or extracted from previous test case response). Now suppose we have 5 test cases and we want to pass incremented value in each test case.
- First we need to write a custom utility method that will increment the input value.
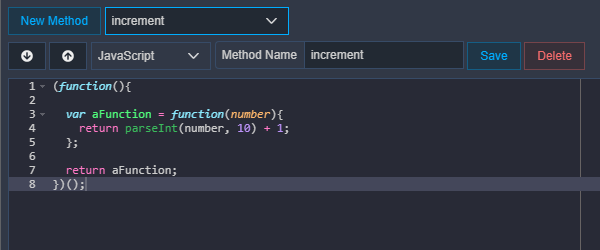
- Then use this utility method in your utility method like below. In the below figure, we have passed the variable "counter" to the utility method "increment".
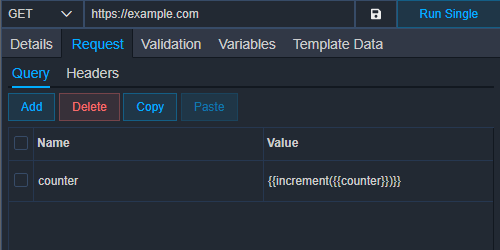
# Importing npm packages in utility methods
To extend the power of utility methods, you may even require npm packages as well in utility methods. For that, you will need to set up the package.json
file in the vREST NG project directory and install the dependencies.
You may view the example vREST NG project which uses an npm package named as faker
in the API test. You may download the project from this Github link (opens new window).
- First define a
package.json
file in your vREST NG project directory with all the dependencies which you want to use in your API tests.
{
"name": "tests-using-npm-packages",
"description": "This vREST NG Project directory explains, how you may use npm packages in your API tests.",
"version": "1.0.0",
"keywords": [
"vREST NG",
"npm",
"utility methods"
],
"dependencies": {
"faker": "^5.4.0"
}
}
In our API tests, we will use this faker
dependency.
- Install the dependencies by using the following command:
npm install
Now you may use this dependency in the utility methods like this:
And then you may use this utility method
FakerLib
in your API tests as usual. You may even invoke nested complex operations using this returned library from utility methods.The above request body will generate the random Request body using the
faker
library as shown in the figure below:
# Writing asynchronous scripts using Promises
With utility methods, you may even perform asynchronous tasks like executing a database query and returning the results. For performing asynchronous tasks, you may use the Javascript Promises (opens new window).
Suppose, you want to execute a database query and return the results of this execution via a utility method. Then you may write the utility method in this way:
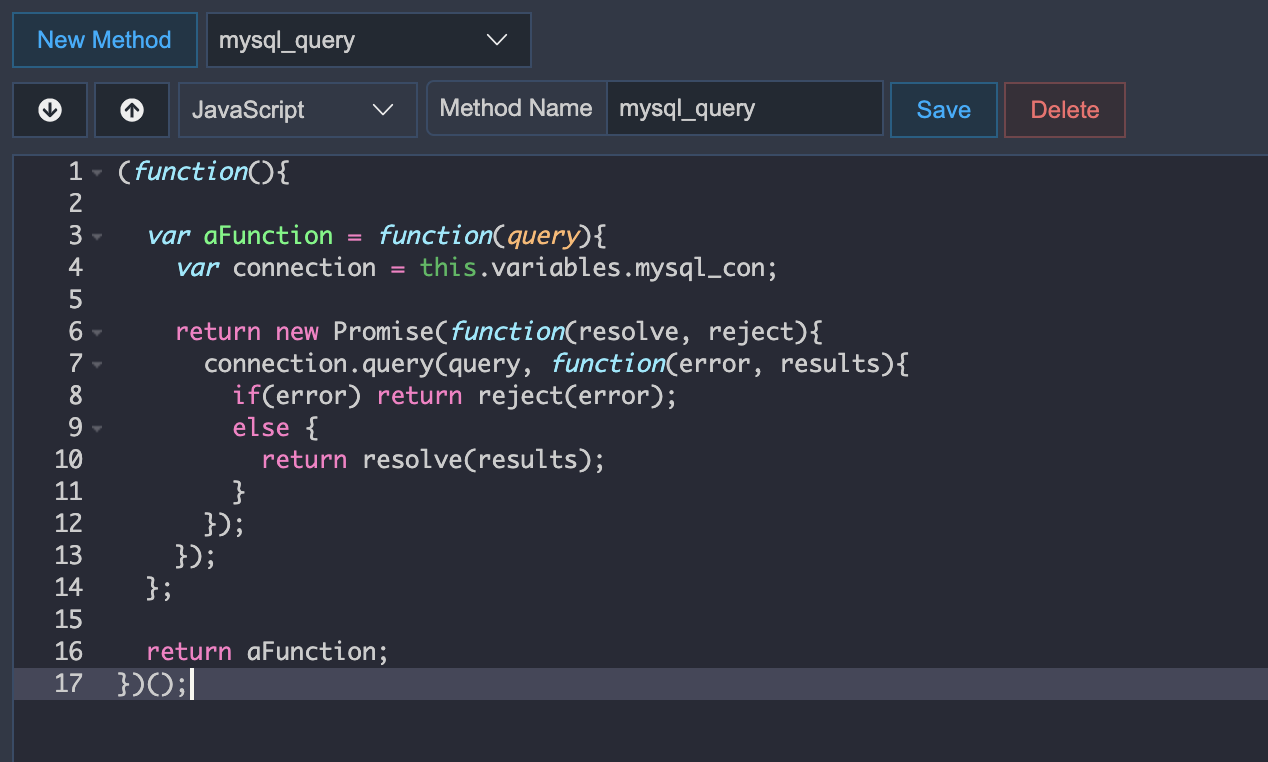
We have used the following code in the above screenshot:
(function(){
var aFunction = function(query){
var connection = this.variables.mysql_con;
return new Promise(function(resolve, reject){
connection.query(query, function(error, results){
if(error) return reject(error);
else {
return resolve(results);
}
});
});
};
return aFunction;
})();
As you can see in the above screenshot that you can return a Promise from the utility method and you may reject any script execution errors and resolve the results on successful executions.